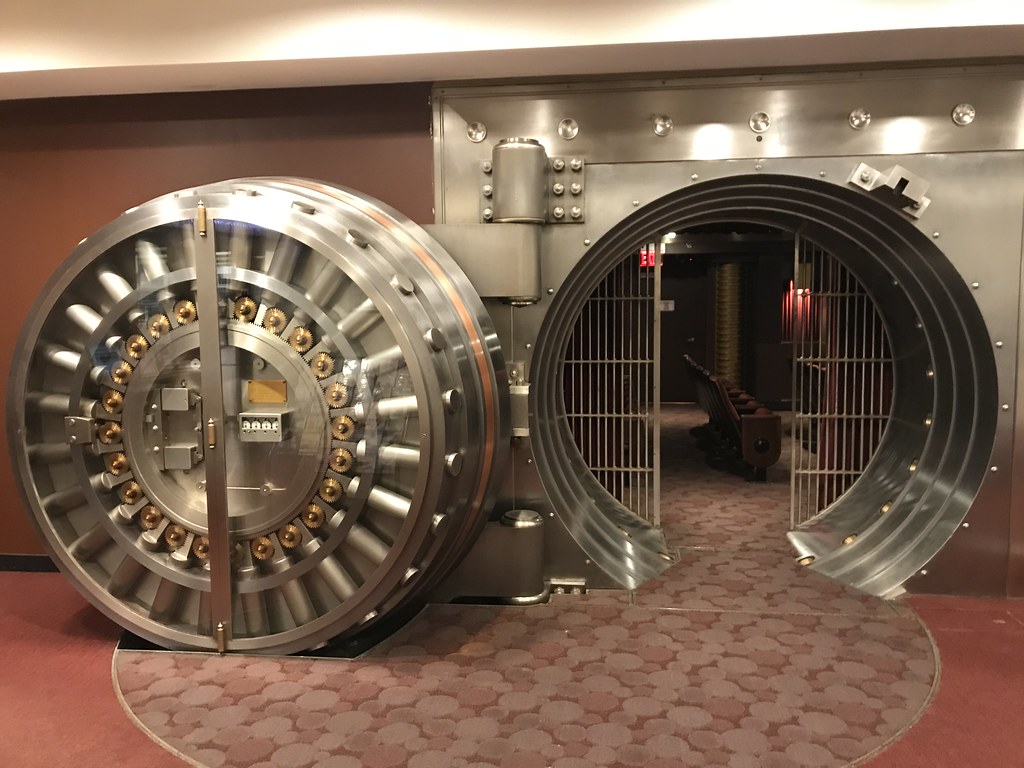
"The film vault" by Sean_Marshall is licensed under CC BY-NC 2.0
Introduction
In the previous article ( Authentication Service Part I), we discussed how we would build the Authentication Service. In this article, we will implement the primary components of the service.Requirements
Before you get started, you will need the following:- Java
- Maven
- Docker
- Docker-Compose
Building the Authentication Service
Additional Infrastructure
As we develop the AuthenticationService, we will need three additional infrastructure elements. A PostgreSQL relational database instance, a PGAdmin-4 PostgreSQL Admin UI, and a RabbitMQ message broker instance. We will deploy both as Docker container images from their respective official Docker Hub repositories. We will add the following to our new Docker-Compose file:loading...
Data Model
The Authentication Service has a simple data model consisting of three tables:- users-Contains user authentication credentials and token related fields.
- roles-Contains a label value pair representing a particular privilege classification.
- users_roles-Provides the many-to-many relationship between Users and Roles.S
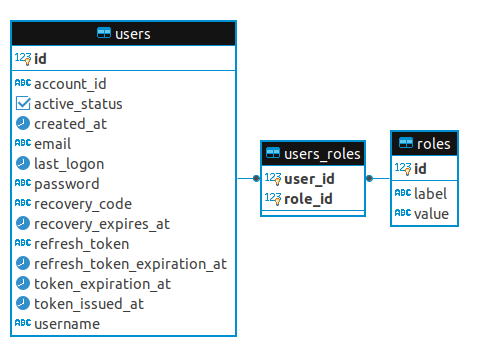
Repositories
The AccountService contains two repositories corresponding to the two entities User and Role.
User Repository
loading...
The User Repository interface extends Spring's CrudRepository class to provide basic persistence methods. These methds are supplemented by service-specfic methods.
Role Repository
loading...
Repository Data Model
Userloading...
Role
loading...
The Role Repository interface extends Spring's CrudRepository class to provide basic persistence methods. These methods are supplemented by service-specfic methods.
Account Event Publisher
The Authentication Service acts as an event-source within the larger application. As service methods are invoked, they publish events to the application's message bus. These include:- Registered
- Authenticated
- Authentication Failed
- Password Changed
- Password Recovery Requested
- Password Recovered
- Account Status Changed
- AccountEventSource- this interface names our outqoing message channel (accountEventChannel) as well as providing a named method to access the message channel (accountEvents()).
loading...
- AccountEventPublisher- this class binds the publisher to the previously defined AccountEventSource.
loading...
loading...
In addition to sending the message event as the message payload, we also set the message header type to ACCOUNT_REGISTERED_EVENT. We use the type header in message listeners to route the appropriate messages to the desired handler methods
JWT Provider
One of the primary responsibilities of the AuthenticationService is the generation of JSON Web tokens. To accomplish this we create the JWTProvider class. This class is responsible for generating a JWT from a User entity and a list of Granted Authorities, as well as providing utility methods to read read :loading...
Email Client Component
An EmailClient component provides integration with the NotificationService Email REST endpoint. This component creates and instance of EmailMessage using it's builder and calls the RestTemplate's postForEntity method to call the endpoint method.loading...
Resources
- AuthenticationService Github repository
- AuthenticationService Docker hub image.
- ThinkMicroservice AuthenticationService Dashboard.
Twitter
Facebook
Reddit
LinkedIn
Email